1. UNIX 标准
- ISO C
- 历史
- ANSI C89
- C99
- restrict
- long long
- 单行注释
- 分散代码与声明
- C11
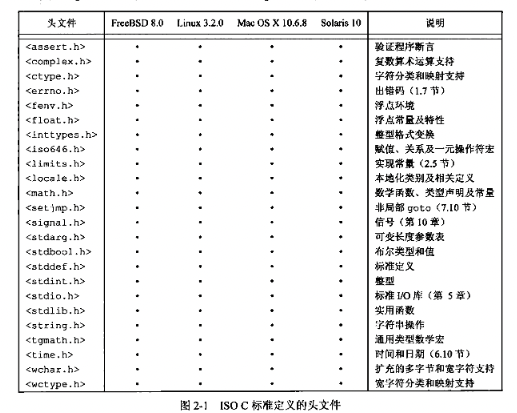
- POSIX
- 提升各种应用程序在Unix系统环境之间的可移植性
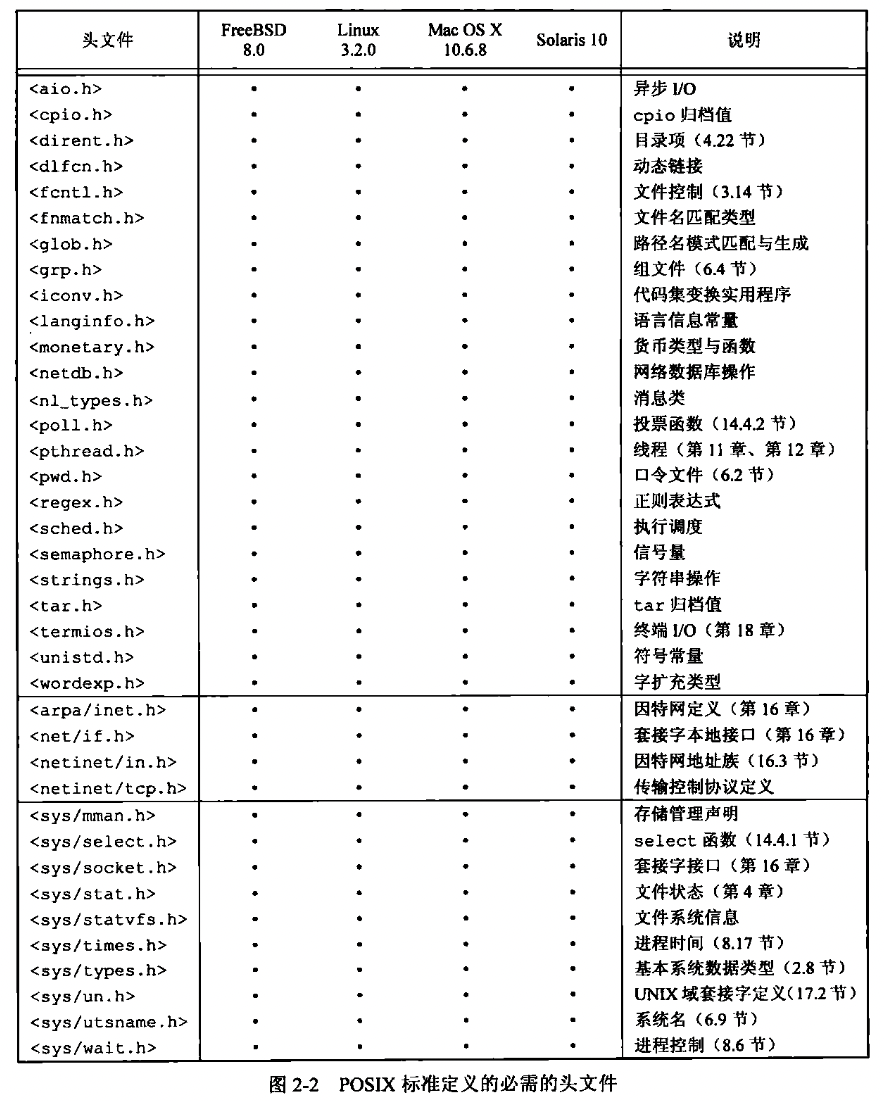
- SUS POSIX超集
- 实现
- FreeBSD
- Linux
- Mac OS X
- Solaris
- 限制
2. 无缓冲IO
- fd (标准输入、输出、标准错误占用了0,1,2)
- 进程有process table entry表,对应了fd 到file pointer的关系(在PCB中存)
- 每个文件描述符表项指向一个已打开文件的指针
- 文件指针指向一个file结构体,如下图
- File Status Flag:只读,读写,写
- 当前读写位置f_pos
- f_count 引用计数(dup、fork等系统调用会导致多个文件描述符指向同一个file结构体)
- f_op 文件函数指针
- f_dentry 指向目录项的指针,即指向/home/akaedu/a的指针,目录项保存inode指针
1 2 3
| #define STDIN_FILENO 0 #define STDOUT_FILENO 1 #define STDERR_FILENO 2
|
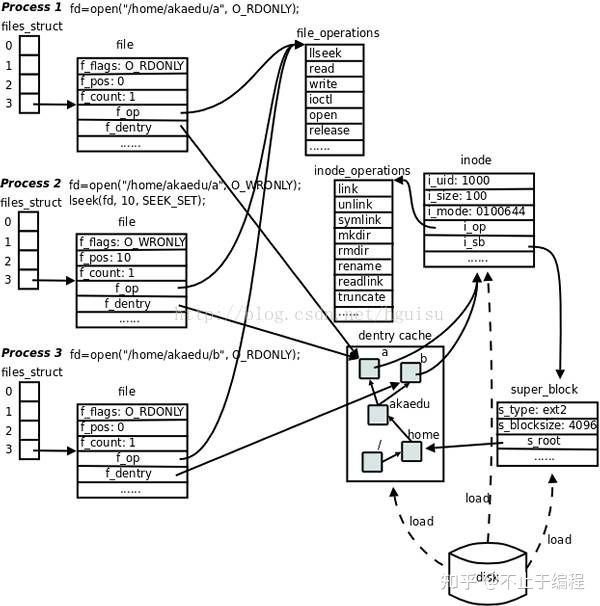
API
open
int open(const char *path, int oflag, /* mode_t mode */);
- oflag
- O_RDONLY
- O_WRONLY
- O_RDWR
- O_APPEND
- O_CLOEXEC 把FD_CLOEXEC常量设置为1(默认为0)
- O_CREAT
- O_EXCL 若同时制定O_CREAT,而文件存在,则出错,若不存在则创建。
- O_DIRECTORY
- O_SYNC 同步写,等IO操作完成
- O_DSYNC 数据同步写,文件属性不同步
- O_TRUNC 截断,长度先为0
1 2 3 4 5 6 7
| result = open(argv[1], O_RDWR); if(result == -1) err_sys("open %s failed\n", argv[1]);
result = open(argv[1], O_RDWR, S_IRUSR | S_IWUSR); if(result == -1) err_sys("open %s with mode S_IRUSR|S_IWUSR failed\n", argv[1]);
|
openat
int openat(int fd, const char *path, int oflag, /* mode_t mode*/)
相对路径
create
创建文件,之前的open无法创建
lseek
1 2 3 4
| #include <unistd.h> off_t lseek(int fd, off_t offset, int whence);
if(lseek(fd, 16384, SEEK_SET) == -1) exit(2);
|
read/write
1 2
| ssize_t read(int fd, void *buf, size_t count); ssize_t wirte(int fd, void *buf, size_t count);
|
pread/pwrite
原子的lseek + read/write
sync/fsync/fdatasync
提交文件系统的cache到disk上
void sync(void)
将data和metadata的cache写到disk
int syncfs(int fd)
将fd对应的data和metadata的cache写到disk,-1表示错误, 0成功
fsync
fdatasync
dup/dup2
创建一个新的fd,是原fd的副本,会打上FD_CLOEXEC的标志。和old fd共同指向同一个file table entry。dup2是原子的。
1 2
| int dup(int oldfd); int dup2(int oldfd, int newfd);
|
fcntl
操纵文件描述符
1 2
| #include <unistd.h> #include <fcntl.h>
|
3. 有缓冲IO
- 无缓冲的IO围绕fd展开: 用户Buffer<->kernel buffer<-> disk I/O
- 有缓冲的IO围绕stream展开: 用户buffer<-> stream buffer <-> kernel buffer <-> disk I/O
流的概念
stream buffer 在刚开始的时候被malloc出来,通过fread,fwrite可以读写,缓冲区满或者可以手动flush将stream buffer写回到kernel buffer。
流的定向:
- 单字节 ASCII byte flow
- 多字节 国际字符集
流缓冲
- 全缓冲 block buffer,最大4096
- 行缓冲 line buffer,最大1024
- 无缓冲
Linux下stdin当不指向交互设备时,全缓冲,指向终端设备,行缓冲。stderr一般是无缓冲的。
FILE 对象
FILE对象维护fd,buffer指针,buffer尺寸,buffer当前字符数,出错标志,等等。
例如
1 2 3 4
| extern FILE *stdin; extern FILE *stdout; extern FILE *stderr;
|
API
流的定向
1 2
| int fwide(FILE *stream, int mode);
|
设置流的行为
1 2 3 4 5 6 7 8 9
|
void setbuf(FILE *stream, char *buf);
void setvbuf(FILE* stream, char *buf, int type, size_t size)
int fflush(FILE* stream);
|
打开
1 2 3 4 5 6 7 8 9 10 11
| FILE* fopen(const char *pathname, const char *type);
FILE* freopen(const char *pathname, const char *type, FILE* fp);
FILE* fdopen(const char *pathname, const char *type);
int fclose(FILE *fp);
|
读写
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| #include <stdio.h>
int fgetc(FILE *stream);
int getc(FILE *stream);
int getchar(void);
int fputc(int c, FILE* stream);
int putc(int c, FILE* stream);
char *fgets(char *str, int size, FILE* stream);
char *gets(char *str);
int fputs(char *str, FILE* stream); int puts(char *str)
|
通用读写
1 2 3
| size_t fread(void *ptr, size_t size, size_t nmemb, FILE* stream); size_t fwrite(void *ptr, size_t size, size_t nmemb, FILE* stream);
|
错误处理
1 2 3
| int feof(FILE* stream); int ferror(FILE* stream); clearerr(FILE* stream);
|
流偏移
1 2 3 4 5 6 7 8 9 10
| int fseek(FILE* fp, long sz, int whence); int fseek(FILE* fp, off_t sz, int whence);
long ftell(FILE* fp); off_t ftello(FILE* fp);
void rewind(FILE *_stream_);
|
格式化输入输出
1 2 3 4 5
| int printf(const char * format ...); int fprintf(FILE *fp, const char* format...); int dprintf(int fd, const char* format...); int sprintf(char *str, ...);
|
标准IO到无缓冲IO的adapter
4. 文件和目录
struct stat
- st_size 文件长度
- st_blksize 文件IO合适的块长度
- st_blocks 分配的合适块数
- st_atime 数据最后访问时间
- st_mtime 数据的最后修改时间
- st_ctime inode数据最后修改时间
- st_mode
- 文件类型
- 普通文件
- 目录文件
- 符号连接
- 块特殊文件
- 字符特殊文件
- FIFO 命名管道
- socket
- 消息队列
- 信号量
- 共享内存
- mode
- set user id bit : S_ISUID
- set group id bit: S_ISGID
- 权限位
文件系统
Linux用VFS(virtual File System)去抽象具体的File system,只存在内存中。
- superblock 文件系统的超级块,保存了文件系统的各个参数
- inode 索引节点,指定一个特定的文件
- denty 目录项,保存子目录、文件的inode信息,维护inode层级关系
- file object
API
状态
1 2 3 4 5 6
| #include <sys/stat.h>
int stat(const char* restrict pathname, struct stat *restrict buf); int fstat(int fd, struct stat *buf);
int lstat(const char *restrict pathname, struct stat *restrict buf, int flag);
|
软链接有自己的inode,存的是路径
权限
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| #include <unistd.h>
int access(const char *pathname, int mode); int faccessat(int fd, const char *pathname, int mode, int flag);
mode_t umask(mode_t cmask);
int chmod(const char *pathname, mode_t mode); int fchmod(int fd, mode_t mode); int fchmodat(int fd, const char *pathname, mode_t mode, int flag);
int chown(const char *pathname, uid_t owner, gid_t group); int fchown(int fd, uid_t owner, gid_t group); int fchownat(int fd, const char *pathname, uid_t owner, gid_t group, int flag); int lchown(const char *pathname, uid_t owner, gid_t group);
|
截断
1 2 3 4
| #include <unistd.h>
int truncate(const char *pathname, off_t length); int ftruncate(int fd, off_t length);
|
链接
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| #include <unistd.h>
int link(const char *existingpath, const char *newpath); int linkat(int efd, const char *existingpath, int nfd, const char *newpath, int flag);
int unlink(const char *pathname); int unlinkat(int fd, const char *pathname, int flag);
#include <stdio.h>
int rename(const char *oldname, const char *newname); int renameat(int oldfd, const char *oldname, int newfd, const char *newname);
#include <unistd.h>
int symlink(const char *actualpath, const char *sympath); int symlinkat(const char *actualpath, int fd, const char *sympath); ssize_t readlink(const char *restrict pathname, char *restrict buf, size_t bufsize); ssize_t readlinkat(int fd, const char *restrict pathname, char *restrict buf, size_t bufsize);
#include <sys/stat.h> int mkdir(const char *pathname, mode_t mode); int mkdirat(int fd, const char *pathname, mode_t mode);
#include <unistd.h>
int rmdir(const char *pathname);
|
硬链接计数和进程计数都为0才可能删除文件
控制相关
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| #include <dirent.h> struct direnct *readdir(DIR *dirp*); DIR *fdopendir(int fd); struct dirent *readdir(DIR *dp); void rewinddir(DIR *dp); int closedir(DIR *dp); long telldir(DIR *dp);
#include <unistd.h> int chdir(const char *pathname); int fchdir(int fd);
#include <unistd.h> char *getcwd(char *buf, size_t size);
|
时间相关
1 2 3 4 5 6
| #include <sys/stat.h> int futimens(int fd, const struct timespec times[2]); int utimesat(int fd, const char *path, const struct timespec times[2], int flag);
#include <sys/time.h> int utimes(const char *pathname, const struct timeval times[2]);
|